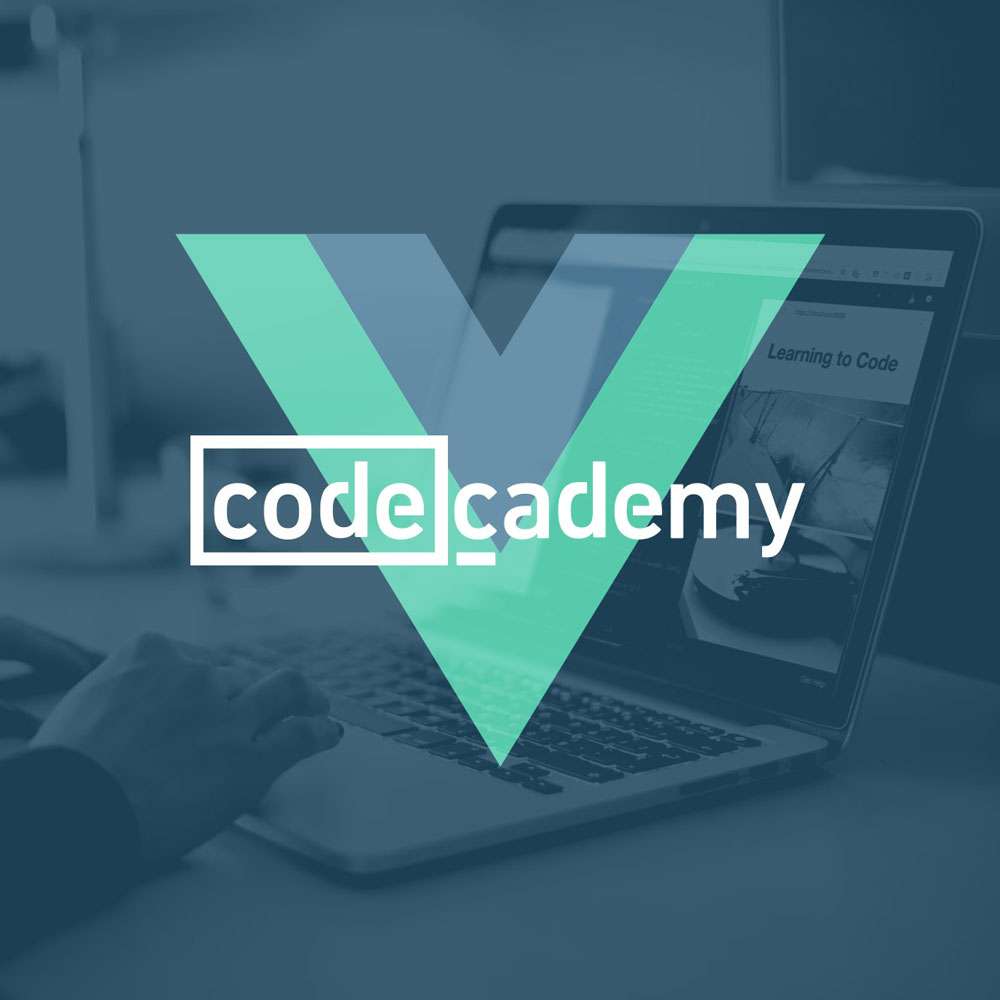
I’m starting to learn Vue.js, and took a course on Codecademy called “Learn Vue.js”. I took notes along the way and compiled them below. The course I took can be found here.
In a script tag, defer means don’t execute the script until the page has loaded. Add the defer attribute to the script tag that includes vue.js. Below is the snippet you can use to get started.
<script src="https://cdn.jsdelivr.net/npm/vue/dist/vue.js" defer></script>
The following code creates a new instance of Vue named “app”.
Vue({}) is called a constructor. Inside the Vue({}) constructor there is only one object – options. Each piece of information the app needs to function is stored within options as a key-value pair.
const app = new Vue({});
Two things you need for your Vue app to function:
- A place to store data
- A syntax for displaying that data
The following is representing #1
const app = new Vue({
username: 'vuetestjoe'
});
The following is representing #2
<p>Hello, {{username}}</p>
When dynamic data is subbed out in the above way, that’s called a template
Directives are snippets of code that perform complex actions but are simplified into easy-to-read bits. A couple examples of directives are described below:
v-if
An if function that will only display the element if the value is true.
<button v-if="userIsLoggedIn">Log Out</button>
v-for
Iterates over a data set and creates an element for every item in that data set
<ul>
<li v-for="todo in todoList">{{ todo }}</li>
</ul>
v-model
Mostly used on forms, the data “username” will be updated when a user modifies that form field.
<input v-model="username" />
When using v-model with multiple checkboxes, the data set must be an array. IF only using one checkbox the data can be a true/false boolean
v-bind
binds a prop in a component to a prop within the main dataset, usually found in app.js or wherever the main Vue statement is. In the example below, I am binding the prop “author” (Found in the tweet component above) to the prop “username” (Found in the main dataset)
<div v-bind:author="username"></div>
v-on
In the following example v-on:click is a directive that says “When this button is clicked, push the value of newTweet to an data set called tweets“
<button v-on:click="tweets.push(newTweet)">Add Tweet</button>
A common shorthand for event handlers involves replacing v-on with @ like so: <form @reset=”resetForm”>
Another example of v-on would be involving forms. In the code below we are saying on reset of the form, run the resetForm method.
<form v-on:reset="resetForm">
Front End Modifiers
Front End Modifiers are properties that can be added to directives to change their behavior. The following code is equivalent to Event.preventDefault(). Similarly, if we had used “.stop” instead of “.prevent”, it would call Event.stopPropagation()
<form v-on:submit.prevent="submitForm">
Component
Basically a chunk of code that is repeatable, like a card or even a footer. Props are the dynamic pieces of data that can be edited within components. The following is an example of a component with a prop named “message”.
const Tweet = new Vue.component('tweet', {
props: ['message', 'author'],
template: '<div class="tweet"><h3>{{ author }}</h3><p>{{ message }}</p></div>'
});
Computed properties
These are not set in data{} but rather in computed{}. They are values that are calculated based on the properties found in data{}. They are usually set as functions. An example can be found below.
const app = new Vue({
el: '#app',
data: {
hoursStudied: 274
},
computed: {
languageLevel: function() {
if (this.hoursStudied < 100) {
return 'Beginner';
} else if (this.hoursStudied < 1000) {
return 'Intermediate';
} else {
return 'Expert';
}
}
}
});
Computed properties can also change the values that are set in data{}. Doing this includes one extra level: get & set. get is the same as just changing a computed property value (shown above) and set is for changing a value in data{}
const app = new Vue({
el: '#app',
data: {
hoursStudied: 274
},
computed: {
languageLevel: {
get: function() {
if (this.hoursStudied < 100) {
return 'Beginner';
} else if (this.hoursStudied < 1000) {
return 'Intermediate';
} else {
return 'Expert';
}
},
set: function(newLanguageLevel) {
if (newLanguageLevel === 'Beginner') {
this.hoursStudied = 0;
} else if (newLanguageLevel === 'Intermediate') {
this.hoursStudied = 100;
} else if (newLanguageLevel === 'Expert') {
this.hoursStudied = 1000;
}
}
}
}
});
data{} is used to store known dynamic data and computed{} is used to store dynamic data that is computed using other pieces of dynamic data
A value inside computed{} will only recompute when a dynamic value used inside of its get function changes.
Watchers
These allow you to make app updates without explicitly using a value in a computed{} function. Watcher functionality is generally used when we do not actually need to continually use the information to compute a new dynamic property, but when we just need to update existing properties when a change happens. Watchers take two parameters: the new value of that property and the previous value of that property. An example of a watcher is below:
const app = new Vue({
el: '#app',
data: {
currentLanguage: 'Spanish',
supportedLanguages: ['Spanish', 'Italian', 'Arabic'],
hoursStudied: 274
},
watch: {
currentLanguage: function (newCurrentLanguage, oldCurrentLanguage) {
if (supportedLanguages.includes(newCurrentLanguage)) {
this.hoursStudied = 0;
} else {
this.currentLanguage = oldCurrentLanguage;
}
}
}
});
It may seem like you could use watch{} in many instances where you could use computed{}. The Vue team encourages developers to use computed{} in these situations as computed{} values update more efficiently than watch{} values.
Methods
The methods{} property is where Vue apps store their reusable functions. The below is an example of a function stored in methods that resets a property in data{}
const app = new Vue({
el: "#app",
data: {
hoursStudied: 300
},
methods: {
resetProgress: function () {
this.hoursStudied = 0;
}
}
});
<button v-on:click="resetProgress">Reset Progress</button>
Input Modifiers
Input modifiers offer quick ways to add logic to directives. The following are a couple examples of input modifiers used on the v-model directive:
- v-model.number – automatically converts the value in the form field to a number
- v-model.trim – removes whitespace from the beginning and ends of the form field value
- .lazy – only updates data values when
change
events are triggered (often when a user moves away from the form field rather than after every keystroke) - .disabled – can be used for form validation. Example below
<button type="submit" v-bind:disabled="!formIsValid">Submit</button>
const app = new Vue({
el: '#app',
computed: {
formIsValid: function() {
return this.firstName && this.lastName && this.email && this.purchaseAgreementSigned;
}
}
});
Inline Styles
v-bind:style can be used to write inline styles. Here’s an example of inline styles with Vue:
<h2 v-bind:style="{ color: breakingNewsColor, 'font-size': breakingNewsFontSize }">Breaking News</h2>
const app = new Vue({
data: {
breakingNewsColor: 'red',
breakingNewsFontSize: '32px'
}
});
If we want to set a value for a hyphenated CSS property, such as
font-size
, we need to put the property name in quotes in order to construct a valid JavaScript object.
A refactor of that above code to simplify it would look like the following:
<h2 v-bind:style="breakingNewsStyles">Breaking News</h2>
const app = new Vue({
data: {
breakingNewsStyles: {
color: 'red',
'font-size': '32px'
}
}
});
v-bind:style can also take an array of style objects as a value. This can be seen in the following code:
const app = new Vue({
data: {
newsHeaderStyles: {
'font-weight': 'bold',
color: 'grey'
},
breakingNewsStyles: {
color: 'red'
}
}
});
<h2 v-bind:style="[newsHeaderStyles, breakingNewsStyles]">Breaking News</h2>
Classes
v-bind can also be used to add classes to elements dynamically. In the example below, we are adding the class “unread” if the “hasNotifications” method evaluates to be true.
<span v-bind:class="{ unread: hasNotifications }">Notifications</span>
.unread {
background-color: blue;
}
const app = new Vue({
data: { notifications: [ ... ] },
computed: {
hasNotifications: function() {
return notifications.length > 0;
}
}
}
You can also add a class in addition to adding a class dynamically based on a method. An example of that is found below. The class “unread” is being added if hasNotifications resolves to true, and then menuItemsClass is defined in data which is another class that is added.
<span v-bind:class="[{ unread: hasNotifications }, menuItemClass]">Notifications</span>
const app = new Vue({
data: {
notifications: [ ... ],
menuItemClass: 'menu-item'
},
computed: {
hasNotifications: function() {
return notifications.length > 0;
}
}
}
Or, you can just add multiple items to the v-bind-class array, such as a value stored in data, and a computed value, and a method – as long as those values evaluate to a CSS class. Below, menuItemClass is a class stored in data, and hasNotifications is a computed value that returns a class based on if there are notifications.
<span v-bind:class="{[menuItemClass, hasNotifications]}">Notifications</span>
Here are 3 different ways to give a <span> the color green:
<span v-bind:style="{ color: 'green' }">I am green</span>
<span v-bind:style="[{ color: 'green' }]">I am green</span>
<span v-bind:style="spanStyle">I am green</span>
const app = new Vue({
data: {
spanStyle: { color: 'green' }
}
});
@joekotlan on X