I recently started learning Vue.js and documented the bits of information that I found particularly useful or helpful along the way.
Although not strictly associated with the MVVM pattern, Vue’s design was partly inspired by it. The variable vm is often used and is short for viewModel.
All Vue components are also Vue instances, and so accept the same options object.
When a Vue instance is created, it adds all the properties found in its data object to Vue’s reactivity system. When the values of those properties change, the view will “react”, updating to match the new values.
Instance Lifecycle Hooks
These are basically points in the Vue app in which you can insert code. Every Vue app goes through stages from setup to final result and at certain points during that process you can tell the app to do certain things. A couple of these hooks are created, mounted, updated, destroyed.
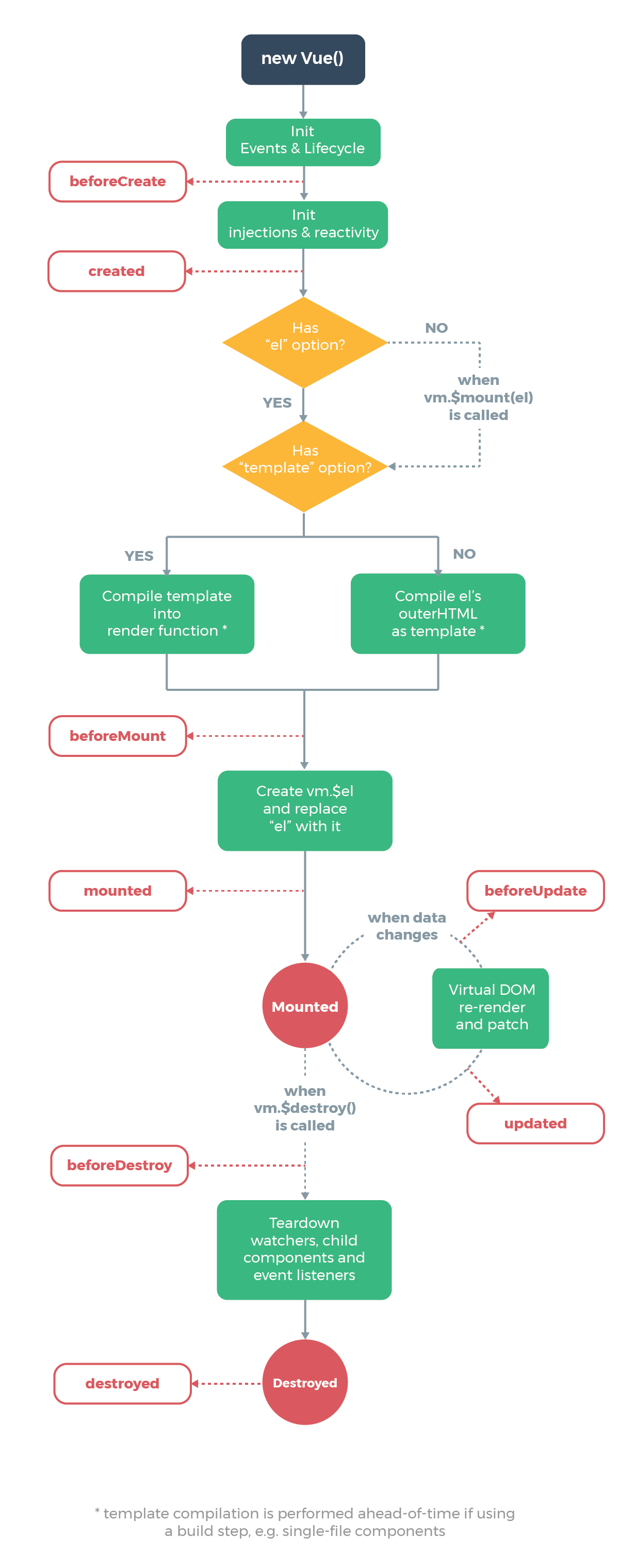
Adding v-once to an element will make sure that the corresponding data does not change within the Vue app. Example below:
<span v-once>This will never change: {{ msg }}</span>
Raw HTML
The double mustaches interprets the data as plain text, not HTML. In order to output real HTML, you will need to use the v-html directive.
<p>Using mustaches: {{ rawHtml }}</p>
<p>Using v-html directive: <span v-html="rawHtml"></span></p>
Using mustaches: <span style=”color: red”>This should be red.</span>
Using v-html directive: This should be red.
Attributes
Mustaches cannot be used inside HTML attributes. Instead, use a v-bind directive:
<div v-bind:id="dynamicId"></div>
In the case of boolean attributes, where their mere existence implies true
, v-bind works a little differently. In this example:
<button v-bind:disabled="isButtonDisabled">Button</button>
If isButtonDisabled has the value of null, undefined, or false, the disabled attribute will not even be included in the rendered <button> element.
The shorthand for v-bind is just a colon, as seen in this example <a :href=”url”> … </a>
When you use a CSS property that requires vendor prefixes in
v-bind:style
, for exampletransform
, Vue will automatically detect and add appropriate prefixes to the applied styles.
@joekotlan on X